https://github.com/apacheli/whirlybird for more details.
Notice: Space has been deprecated in favor of a newly reworked library. Check out
Space
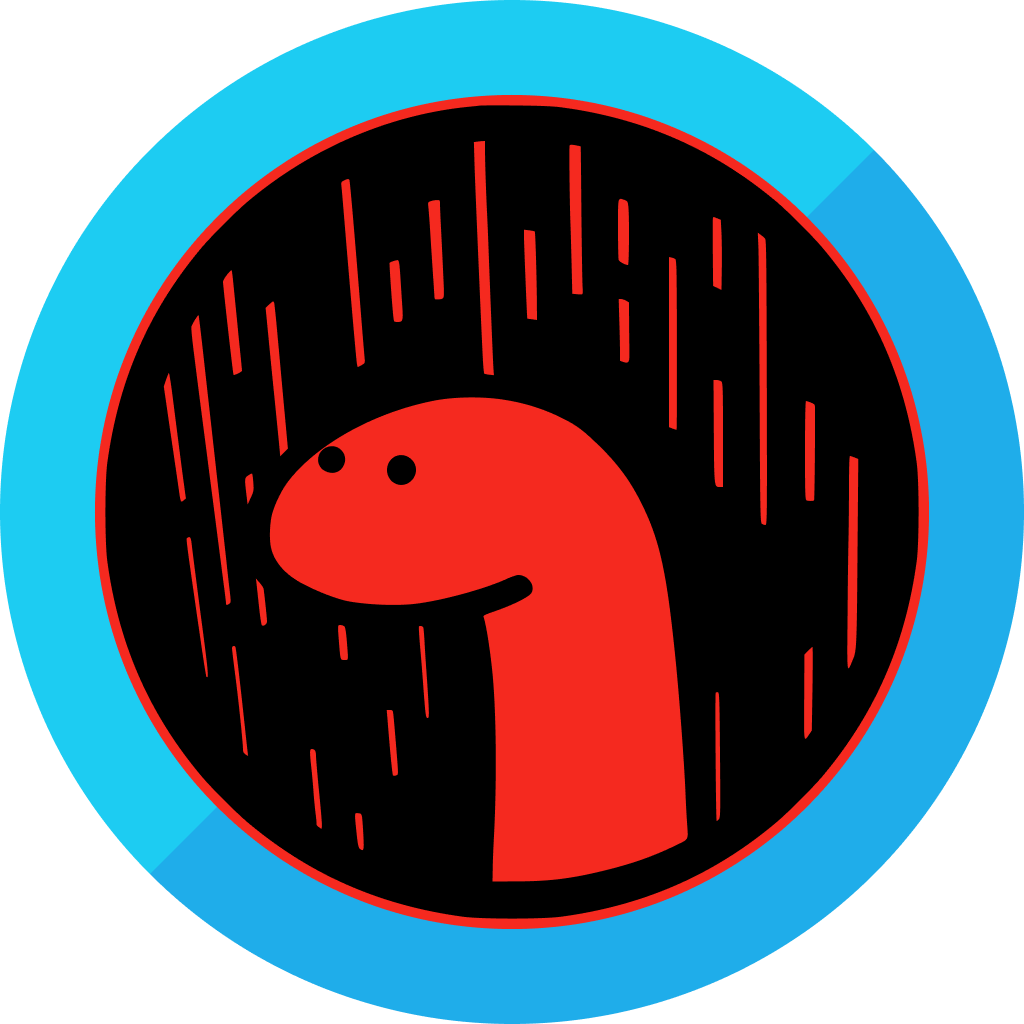
Space is currently under heavy development and is therefore highly unrecommended for production. Any contributions are greatly appreciated. The contributing guide contains further instructions.
Space is a collection of fast, scalable, low-level Deno modules for interacting with the Discord API.
Table of Contents
Install
Each module must be imported individually. For most end-users, importing the
client
module should be sufficient. For more proficient users,
the core modules are public and available for use. They can be viewed
here.
The Deno manual provides a more in-depth explanation about dependency management in Deno.
export * from "https://deno.land/x/space@0.11.0-alpha/core/client/mod.ts";
The release notes contains all available versions.
Latest (not recommended for production):
export * from "https://github.com/apacheli/space/raw/dev/core/client/mod.ts";
Getting Started
Run an example directly from the CLI:
deno run --allow-env --allow-net https://deno.land/x/space@0.11.0-alpha/examples/example.ts
More examples are available here.
A simple program using Space to power a Discord bot:
import { Client, GatewayIntents } from "./deps.ts";
const token = Deno.env.get("BOT_TOKEN") ?? prompt("bot token:");
if (!token) {
throw new TypeError("An invalid bot token was provided.");
}
const client = new Client(`Bot ${token}`);
(async () => {
for await (const [message, shard] of client.gateway.onMessageCreate()) {
if (message.content === "!ping") {
client.http.createMessage(message.channelId, {
content: "pong!",
});
}
}
})();
client.gateway.connect({
intents: GatewayIntents.GuildMessages,
});
To run it:
deno run --allow-env --allow-net main.ts
The module reference is available at the generated documentation.
Resources
Come hang out with us at the Space Discord server! All participating members must abide by the terms of the Space code of conduct.